PostgreSQL provides some statements that assist users in decision-making scenarios. These statements take a decision based on some specific criteria/condition and are hence referred to as control statements. In Postgres, the if-statement is one of the most valuable and frequently used control statements. It executes only those expressions that satisfy the specified condition. The below flow charts demonstrate the workflow of the Postgres IF statement:
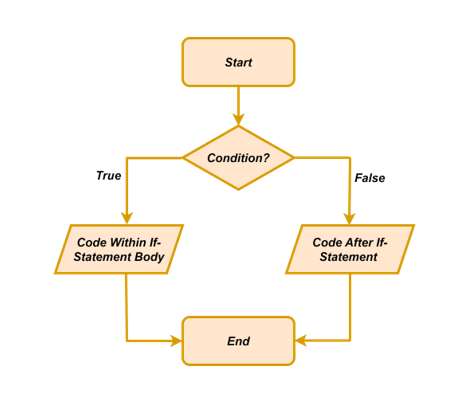
Now, let’s explore the use of PostgreSQL if-statement with examples.
How to Use IF Statement in PostgreSQL?
In Postgres, the IF statement only treats the true condition. The syntax of the IF statement is as follows:
IF condition THEN statement(s); END IF;
Here, the “condition” represents a criterion that must be satisfied to execute the “statements” specified within the THEN block.
Important: If an expression doesn’t satisfy the specified criteria, then the if-statement moves the control to the next statement.
Example 1: How Does the IF Statement Work in PostgreSQL?
Let’s consider a scenario to understand the working of the IF statement:
DO $$ DECLARE user_age INT := 25; BEGIN IF user_age > 18 THEN RAISE NOTICE 'Access Granted'; END IF; IF user_age < 18 THEN RAISE NOTICE 'Access Denied'; END IF; RAISE NOTICE 'Control Shifted Out of IF Statement'; END $$;
- Firstly, we utilized the DO statement which indicates that we are going to execute all the statements that come under it.
- Next, we declared a variable user_age and assigned it a value of 25.
- After that, we utilized the BEGIN statement to start the execution.
- Next, we utilized the IF statement that checks if the “user_age > 18”. If yes, then show the message “Access granted”.
- If no, then the control will be moved to the next statement after the first END IF; where the variable’s value will be tested against the second condition i.e. “user_age < 18”.
- Once done with IF conditions, the control will be moved to the next statement specified after the “END IF” statement.
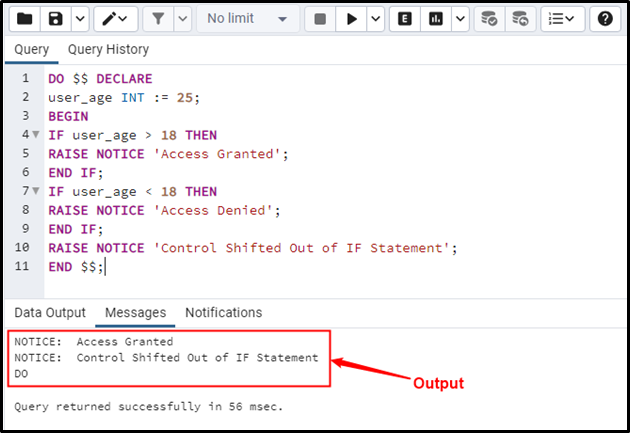
Let’s modify the variable’s value and see how the if-statement works in that case:
user_age INT := 15;
This time the variable’s value will be tested against the first condition i.e. user_age > 18.
- The if-statement will return false, so the statements associated with the first condition will be skipped.
- The control will be moved to the second condition i.e. user_age<18.
- The if statement will compare the user’s age with the specified condition, i.e. 15 < 18. Consequently, it will return the following output:
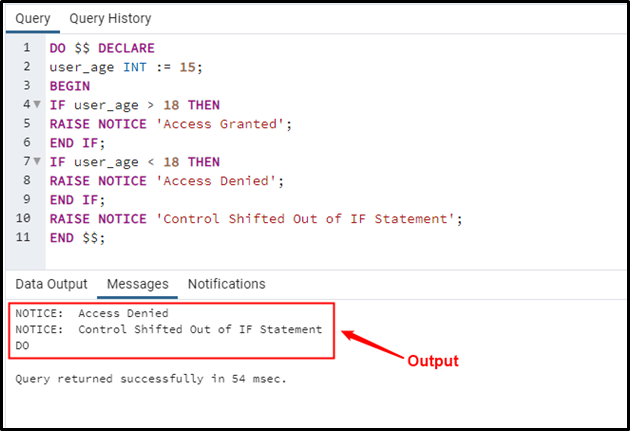
The notice “Access Denied” authenticates the working of the IF statement.
Example 2: IF Statement With False Condition
As discussed earlier, the Postgres IF statement works perfectly fine only with true conditions, however, it doesn’t deal with false conditions. For instance, in the following code block, none of the specified conditions are true; let’s see how the IF statement deals with it:
DO $$ DECLARE user_age INT := 18; BEGIN IF user_age > 18 THEN RAISE NOTICE 'Access Granted'; END IF; IF user_age < 18 THEN RAISE NOTICE 'Access Denied'; END IF; RAISE NOTICE 'Control Shifted out of IF Statement'; END $$;
This time,
- We initialize the variable “user_age” with a value “18”.
- In the BEGIN block, the “user_age” will be checked against both IF conditions. The specified age doesn’t satisfy any of the IF statements. So, the control will be moved to the next statement specified after the “END IF” statement.
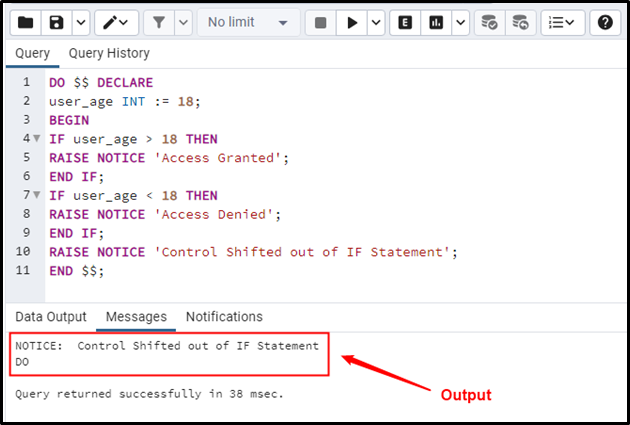
The output confirms that the IF statement doesn’t deal with the FALSE condition, instead, it simply moves the control to the next statement.
Example 3: Dealing With False Conditions
From the above examples, we can conclude that it doesn’t matter how many IF statements we use, they only deal with true conditions, and they have nothing to do with the FALSE conditions. So, the question is how to address the false conditions. Well! The IF-ELSE statement comes in handy here. The below syntax will help you understand this concept better:
IF condition THEN --Executes if condition returns TRUE statements; ELSE --Executes otherwise (FALSE condition) alternative-statements; END if;
Let’s modify the code of example 3 by embedding the ELSE condition in it:
DO $$ DECLARE user_age INT := 18; BEGIN IF user_age > 18 THEN RAISE NOTICE 'Access Granted'; END IF; IF user_age < 18 THEN RAISE NOTICE 'Access Denied'; ELSE RAISE NOTICE 'Try Again'; END IF; RAISE NOTICE 'Control Shifted out of IF Block; END $$;
This time the “FALSE” condition is successfully tackled using the ELSE condition, as shown in the following output:
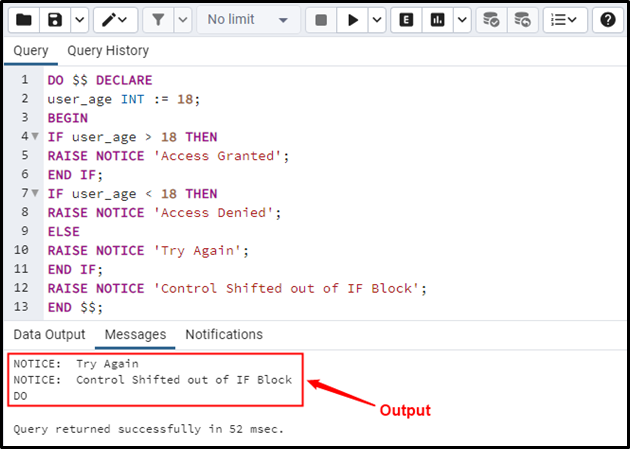
To learn more use cases of the ELSE condition, go through the following dedicated guide on IF-ELSE.
Example 4: Dealing With Multiple Conditions
Use the IF-THEN-ELSIF instead of a simple IF-ELSE if you have to evaluate multiple conditions. In such a case, if the specified condition is true, the associated statement within that branch will be executed. If all the specified conditions are evaluated to FALSE, then the control will be moved to the “else” branch, and the associated statements within it will be executed.
Here is how you can implement IF-THEN-ELSIF in Postgres:
IF condition1 THEN statement1; ELSEIF condition2 THEN statement2 ... ELSEIF conditionN THEN statementN; ELSE statement; END IF;
Here, the “TRUE” conditions will be tackled by the “IF” statement, and the false conditions(that don’t satisfy the IF statement) will be addressed by ELSEIF. While the conditions that neither meet the IF statement nor the ELSEIF statement will be addressed by the ELSE block.
Here is a code example that demonstrates how multiple false conditions can be addressed using the “IF-THEN-ELSIF” statement:
DO $$ DECLARE emp1_salary INT := 55000; emp2_salary INT := 54000; BEGIN IF emp1_salary < emp2_salary THEN RAISE NOTICE 'emp1_salary is less than emp2_salary'; ELSIF emp1_salary > emp2_salary THEN RAISE NOTICE 'emp1_salary is greater than emp2_salary'; ELSE RAISE NOTICE 'emp1_salary is equal to emp2_salary'; END IF; END $$;
In this example, the salaries of two employees are compared, and a notice is raised accordingly. In the considered example, the condition specified in the ELSIF block is evaluated as TRUE, so the notice/message associated with that block is retrieved in the output:
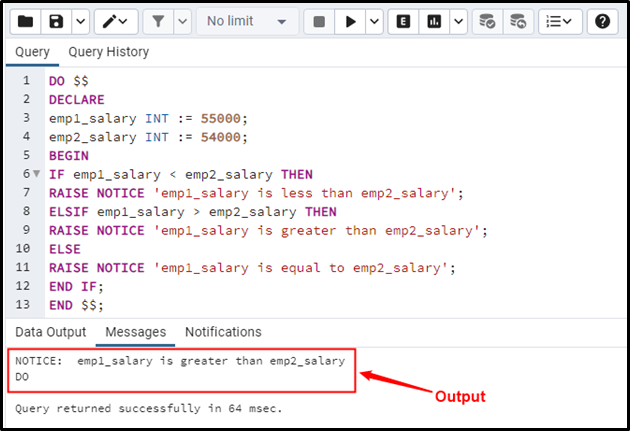
To learn more about the usage of ELSE IF in Postgres, read our dedicated guide on PostgreSQL Else If Statement.
Conclusion
In PostgreSQL, the if-statement is one of the most valuable and frequently used control statements. The If statement executes only those expressions that satisfy the specified condition. If an expression doesn’t satisfy the specified criteria, then the if-statement moves the control to the next statement. This post considered several examples to explain the working of the if statement in Postgres.