The term “conditional select” refers to data selection based on some particular conditions. In PostgreSQL, conditional statements, such as the IF ELSE and CASE, are used to define the selection criteria. However, the Postgres IF ELSE statement can’t be utilized within the SELECT query. Therefore, to make a conditional selection the SELECT statement must be executed with the CASE statement.
This write-up will guide you on how to perform the conditional selection in Postgres.
PostgreSQL Conditional Select With Examples
In PostgreSQL, the CASE statement lets us check multiple conditions and retrieves the results based on the specified conditions. While the SELECT statement allows us to fetch the table’s data. Use the following syntax to use the CASE expression within the SELECT statement:
SELECT col_list, CASE WHEN cond_1 THEN result_1 WHEN cond_2 THEN result_2 … WHEN cond_n THEN result_n ELSE else_result END FROM tab_name;
In the above-given syntax:
- cond_1, cond_2, …, cond_n represents the conditions based on which the reult_1, result_2, …, or result_n will be selected.
- For instance, when the cond_1 is true, the result_1 will be retrieved.
- If no condition is true, the result specified within the ELSE statement will be retrieved.
- tab_name represents the table from which the data will be selected.
In simple terms, the above syntax will let you select the table’s data based on some specific conditions.
Example: Understanding Conditional Select
A table named “bikes_info” has already been created with the following data:
SELECT * FROM bikes_info;
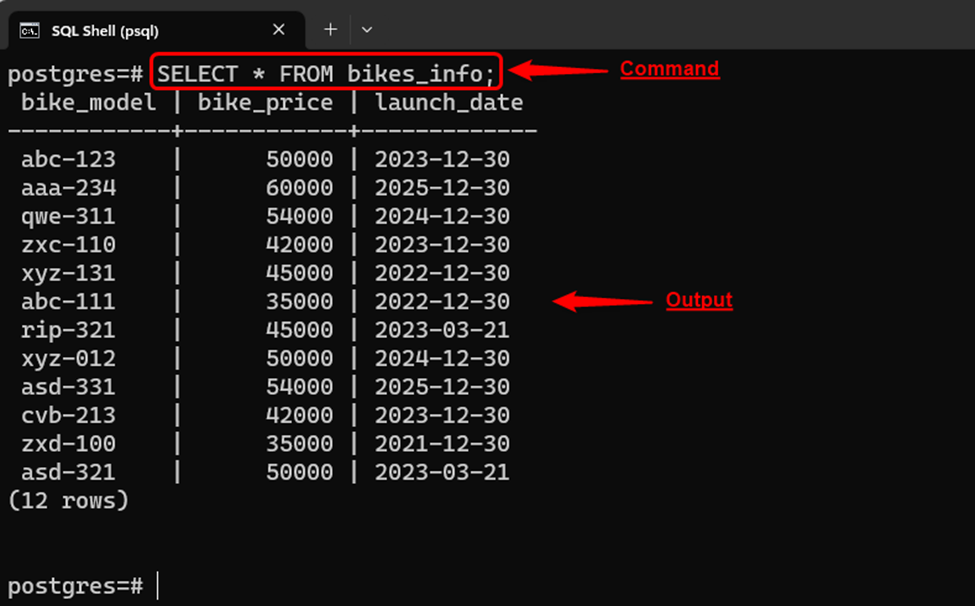
In the following snippet, the CASE statement is used within the SELECT statement to display the results based on the conditional select:
SELECT bike_model, bike_price, launch_date, CASE WHEN launch_date BETWEEN '2020-01-01' AND CURRENT_DATE THEN 'Already Launched' WHEN launch_date BETWEEN CURRENT_DATE AND '2024-01-01' THEN 'Launching Soon' WHEN launch_date > '2024-01-01' THEN 'Will be launched in Future' ELSE 'Already Launched' END CASE FROM bikes_info;
In this example program:
- Columns to be selected are specified in the SELECT statement.
- The CASE statement is also used in the SELECT statement to make the conditional selection.
- The selection criteria are defined using the WHEN clause. While the corresponding results are specified using the THEN clause.
- The selection condition is defined using the “launch_date” column of the “bikes_info” table.
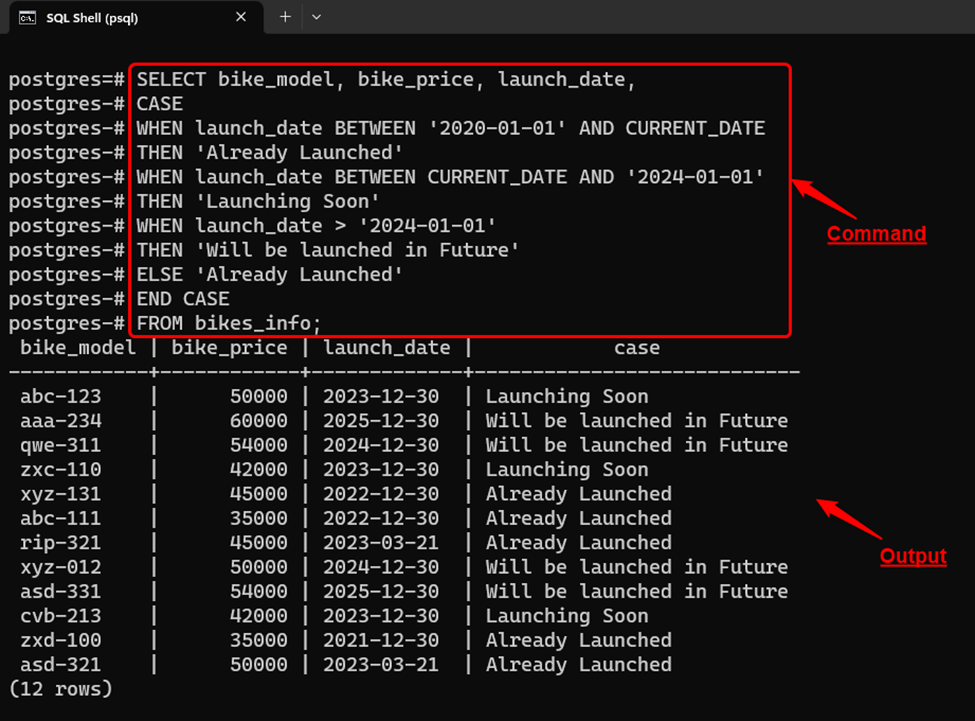
The above snippet demonstrates how to do conditional selection in PostgreSQL.
Conclusion
To make the conditional selection in PostgreSQL, the CASE expression must be used within the SELECT statement. In PostgreSQL, the SELECT statement allows us to fetch the table’s data. While the CASE statement lets us check multiple conditions and retrieves the results based on the specified conditions. The conditions can be defined using the WHEN clause and the corresponding results can be specified using the THEN clause. This post presented a detailed guide on conditional selection in PostgreSQL using examples.